Hello and Welcome to Python Programming for beginners, where we teach you Python from Scratch. In the last class, we saw Object Oriented Programming or OOPs in python and now we are heading towards our journey as a python developer, If you haven’t checked OOPS out, CLICK HERE. So, on that note, Today’s topic is Inheritance in Python.
Inheritance in Python
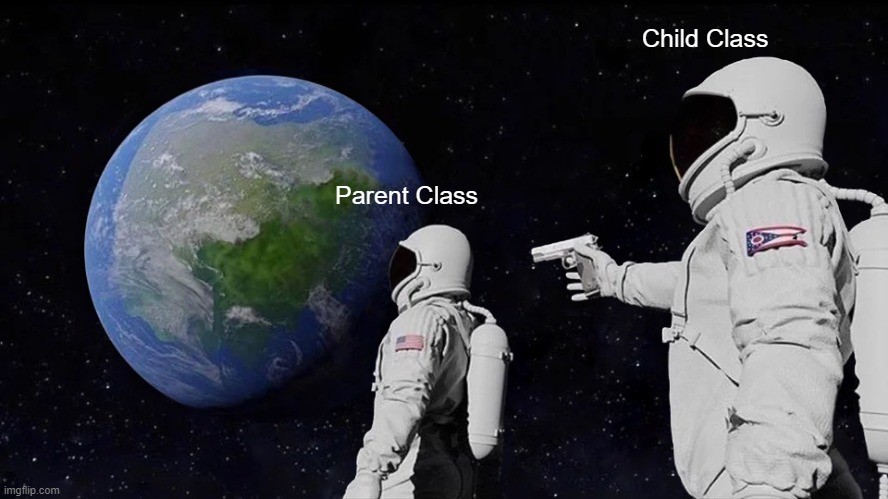
Inheritance is a way of creating a new class from an existing class. The syntax goes like:
Class Employee:
#code
Class Programmer(Employee):
#code
Here the Class Employee is the Base class or the Parent class and the Class Programmer is the derived class or the child class. Taking the parent-child analogy, like a parent gene or characteristics is inherited by the child, same in Inheritance this happens. The Child class uses or inherits the Parent Class.
We can use the methods and attributes of Employee in Programmer object. Also, we can overwrite or add new attributes and methods in the Programmer class. Let’s see an example:
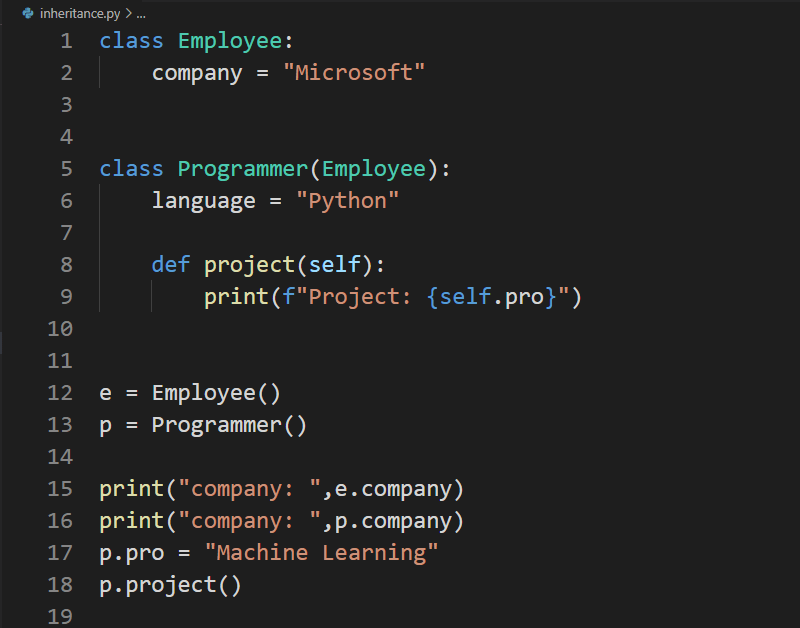
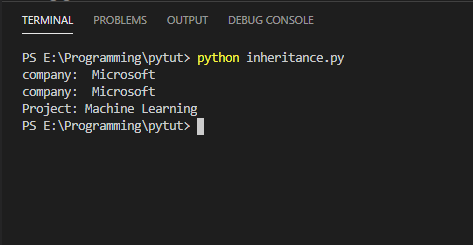
Here we make two classes Employee and Programmer with their attributes and method. Then we instantiate the objects. And all that you know, the main catch here is when we try to access p.company, when there is no company attribute in programmer, but it did print Microsoft because we are using Employee class as our parent class, so we can use its attributes.
Types of Inheritance
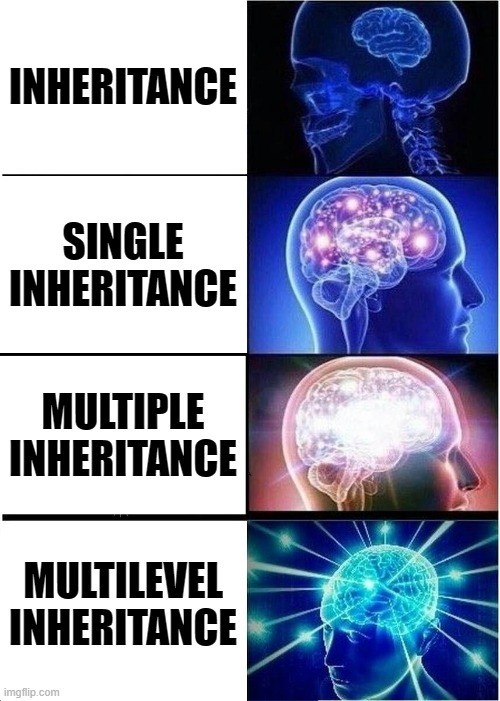
- Single Inheritance – Single Inheritance occurs when the Child class inherits only a single parent class. Like the example used above.
- Multiple Inheritance – Multiple Inheritance occurs when the child class inherits from more than one parent class. Ex:
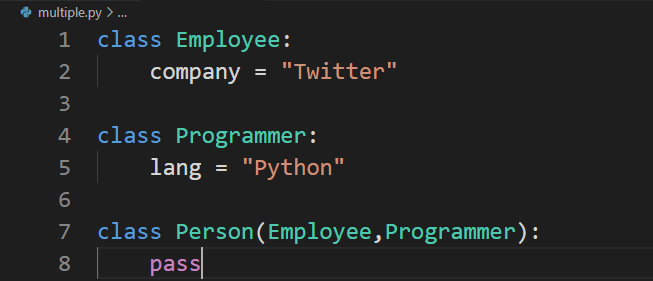
- Multilevel Inheritance – When a child class becomes a parent class for another Child class. Ex:
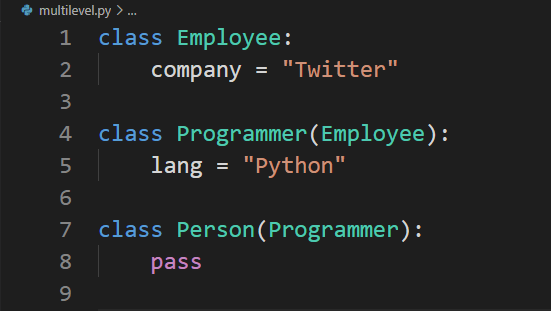
Super() Methods
Super Methods is used to access the methods of a super class in the derived class. Syntax:
Super().__init__() – It calls the constructor of the base class.
Class methods – A class method is a method that is bound to the class and not the object of the class.
@classmethod decorator is used to write a class method.
@classmethod
Def (cls, p1, p2):
Pass
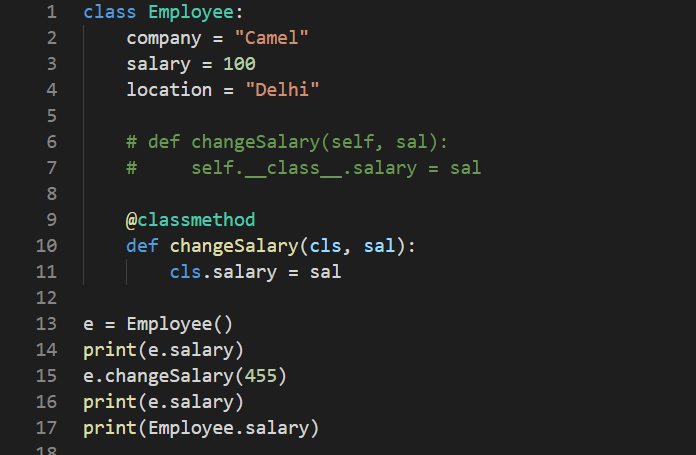
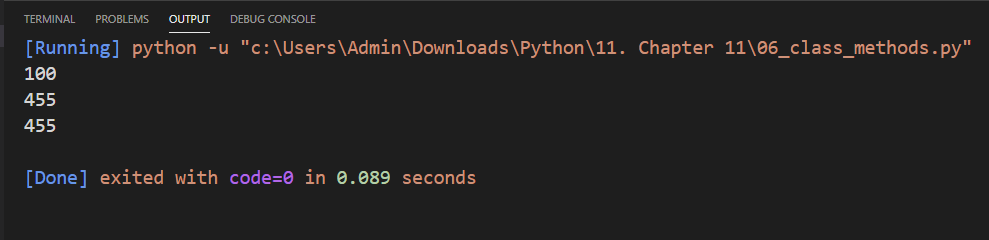
@property decorators – Consider the following class:
Class Employee:
@property
def name(self):
return self.ename
if e = Employee() is an object of Class employee, we can print e.name to print the ename/call name function. It just makes the method look like a property of the attribute.
@getters and @setters – The method with @property decorator is called a getter method.
We can define a function + @name.setter decorator like this:
@name.setter
def name(self,value):
self.ename = value
Operator Overloading in Python
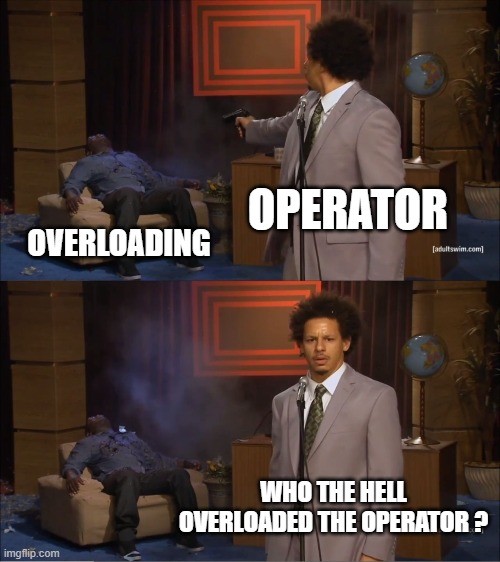
Operators in python can be overloaded using dunder method(with two underscore like __init__). These methods are called when an operator is used on the objects. Like:
- p1 +p2 – p1.__add__(p2)
- p1 – p2 – p1._sub_(p2)
- p1 * p2 – p1.__mul__(p2)
- p1 / p2 – p1.__truediv__(p2)
- p1 // p2 – p1.__floordiv__(p2)
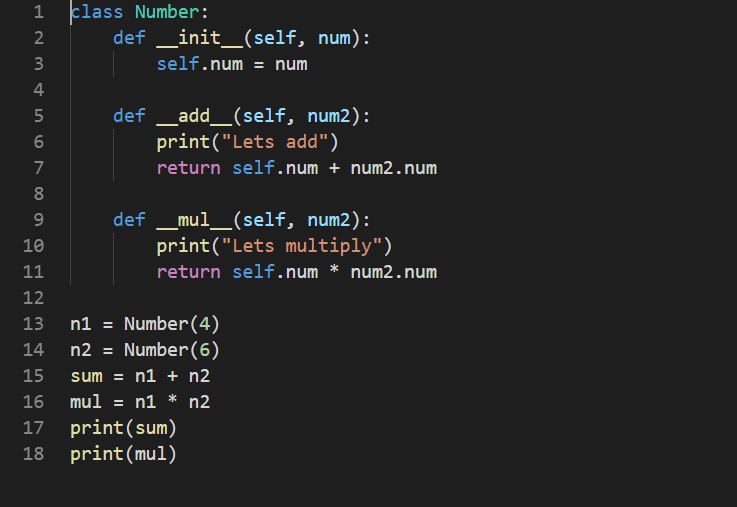

Other dunder methods in Python
- __str__() – used to set what gets displayed upon calling str(obj).
- __len__() – used to set what gets displayed upon calling __len__() or len(obj)
So on that note, that’s it for today, if you want to know more, CLICK HERE. Let’s have a revision of what we learned today we saw Inheritance, Types of Inheritance,Super method, Class methods, @property decorator, @getters and @setters, Operator Overloading, and dunder methods. So, that’s it for today, this is Dolores Haze, signing off, I will meet you in next one, till then “Happy Coding” and Bye.