Welcome to another class of Python Programming guys, where we teach you Python programming from Scratch. In the last class, we see a lot of stuff from keywords to variables. Data Types and typecasting and how things work in a Python program. So let’s started with today’s class. Today we are going to learn about Operators.
What are Operators in Python?
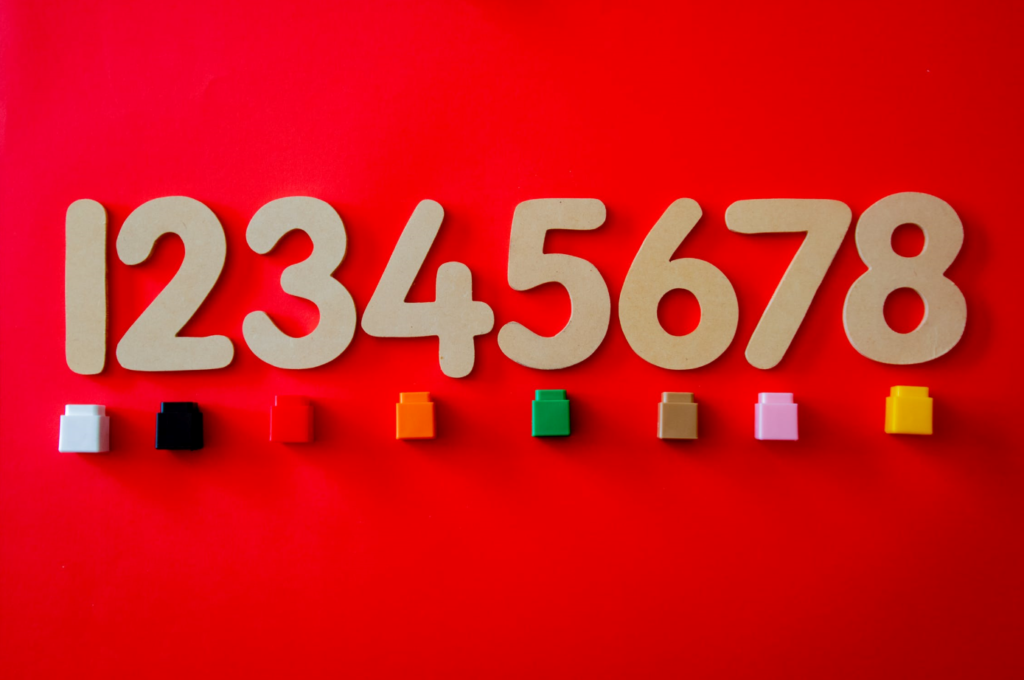
Operators are symbols that are used for performing certain specific tasks. Operators can work either on a single variable or more than one variable. The variables or values on which these operators work are known as operands.
Types of Operators
There are 7 types of major operators in Python. Let’s see what these are and what they are used for.
- Arithmetic Operator: As the name suggests, These types of operators are used to perform arithmetic operations. Like one we see in the adding two numbers program in the previous chapter. This includes all major arithmetic symbols like:
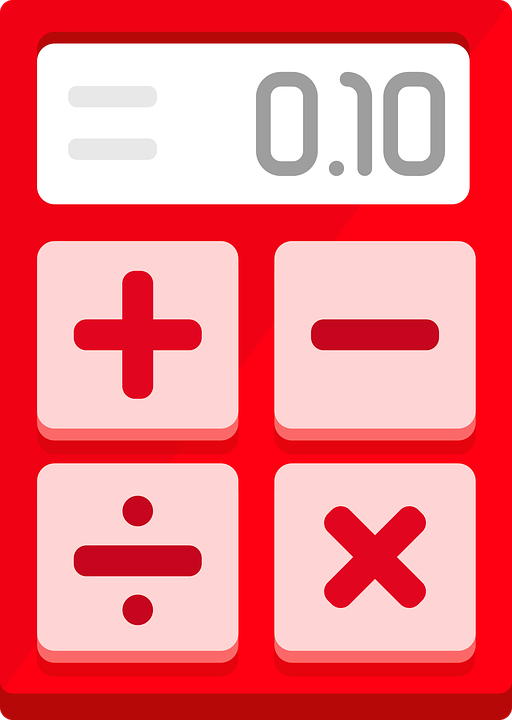
Sum or plus: “+”
Subtract or minus: “-“
Multiply: “*”
Division: “/”
Modulus (For finding Remainder): “%”
Ex: a + b, a – b, a*b, a/b, a%b.
- Assignment Operator: Assignment operator is used to assign values to variables like we have seen before. This operator symbol is “=”.
Ex: a = 10 or b = 45
- Comparison Operator: Comparison operators are used to compare the value of a variable if its equal to the value or not equal to or greater or lesser. It uses symbols such as:
a == 5 -> Here this is a == 5, not a = 5, a =5 is an assignment operator which assign the value 5 to variable a but here “==” is checking if the value of is 5 or not, it is comparing the value assigned to a to value given, if it is then it is true otherwise false. Many beginners do mistakes in these two things over assignment and comparison “==” operator.
a > 5 or a < 5 -> Here, the greater and lesser than signs are checking if a is greater or lesser than 5 respectively.
a > = 5 or a < = 5 -> This thing is similar to above one, except the equal to sign. Here, Unlike the above statement, it will also check a = 5 with greater or lesser than signs.
a != 5 -> It is checking if a is not equal to 5. “!” indicates not, it is totally opposite of the first condition, where we were checking if a == 5. But here we are checking if a is not equal to 5 instead.
- Logical Operator: Logical works on Boolean Algebra with “AND”, “OR” and “NOT”.
Ex: a and 5; b or 10.
- Identity Operator: These types of operators return “TRUE” or “FALSE” according to a comparison of two variables. It uses “is” and “is not” symbols.
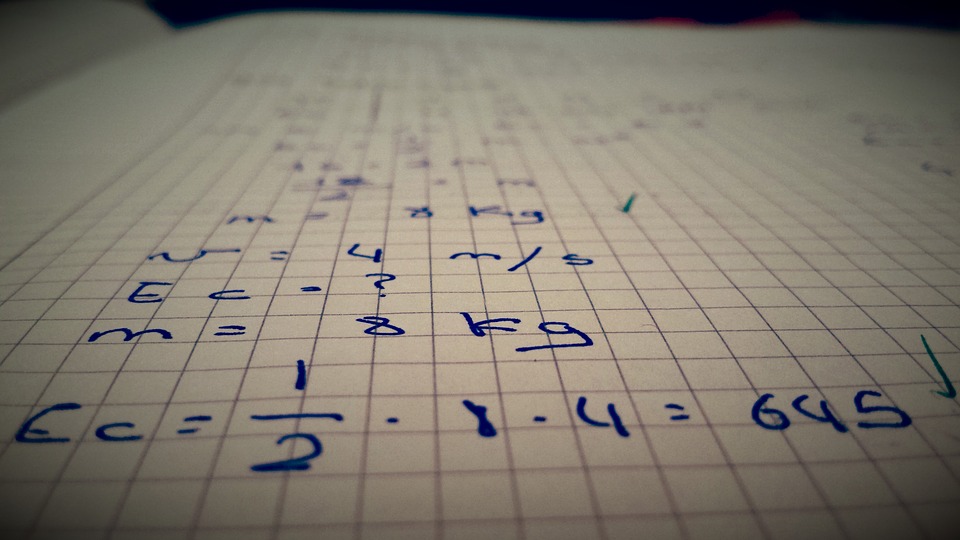
Ex: a is b or a is not b -> It will return TRUE or FALSE accordingly.
- Membership Operator: This operator checks a particular element in a list. Suppose, if a class teacher wants to check if your name is in the class list or not. It will simply use the Membership Operator as It checks the Membership of an element in the list. It uses “in” and “not in” symbols.
- Ex: Void in Class or Void not in class.
- Bitwise Operator: As the name suggests bitwise operators work on Bits or in Binary value or base 2. It uses 0s and 1s as symbols to work.
Ex: 0 – 00
1 – 01
2 – 10
3 – 11
This shows different numbers with their binary values. For Binary you can use “|” for OR and “&” for AND.
That’s all for today, Today we have learned about different operators and how to use them in your programs. If you haven’t checked out the previous posts go check them out HERE. This is Dolores Haze, signing off, I will be there for you in the next class. Till then, “Happy Coding”.