Hello and Welcome to Python Programming for beginners, where we teach you Python from Scratch. In the last python class, we learn about List and Tuples in Python and how to use them in our programs. If you haven’t check them out yet, CLICK HERE. If you have let’s move ahead.
Today we are continuing with our python programming class and the topic of today’s class is Sets and Dictionary in python.
What are Sets in Python?
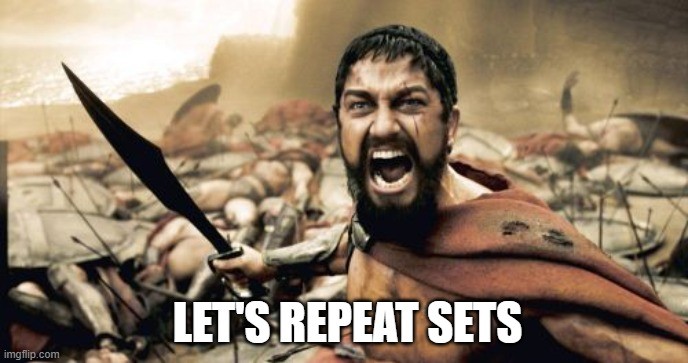
Sets are a collection of non-repetitive elements.
If you are from a Maths background, you know what sets are, if you are not, then don’t worry. It’s simple, the major standing point of sets data type is there are no repetitive elements. Even if you try to enter one element twice or thrice, it ignores it. Sets don’t allow duplicate. Let me show you how.
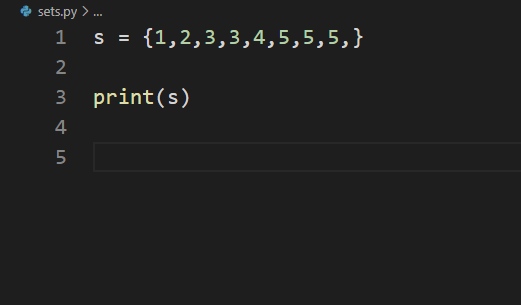
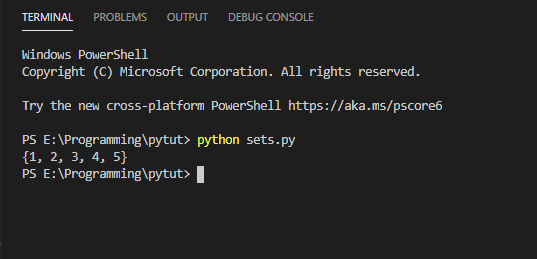
Here we enter a Set named “s”, with values repeating, but when we print the set, we got { 1, 2, 3, 4, 5 } with non-repeating values.
Properties of Sets
- Sets are unordered. That means the element’s order doesn’t matter.
- Sets are unindexed. That means we cannot access sets using indices like we used to do in Lists.
- There is no way to change items in sets.
- Sets cannot contain duplicate values.
Operations on Sets
S = {1, 8, 2, 6}
- Len() – The len() function returns the length of the set.
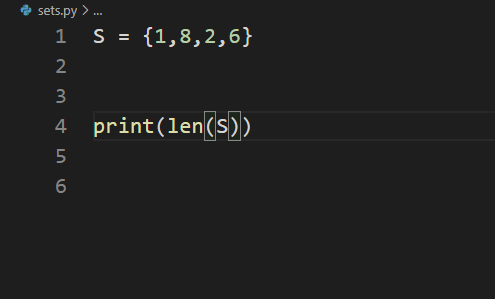
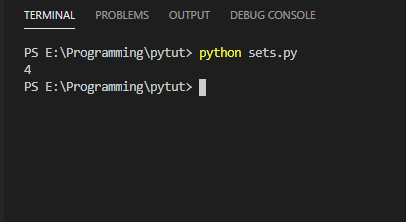
- Set.remove() – It updates the set and removes the element passed to the remove function.
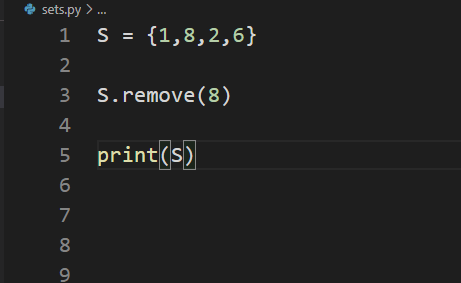
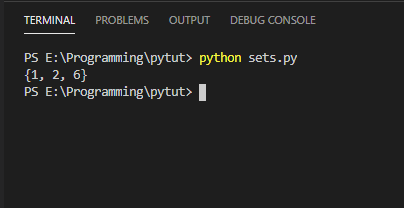
- Set.pop() – Removes an arbitrary element from the set and returns the element removed.
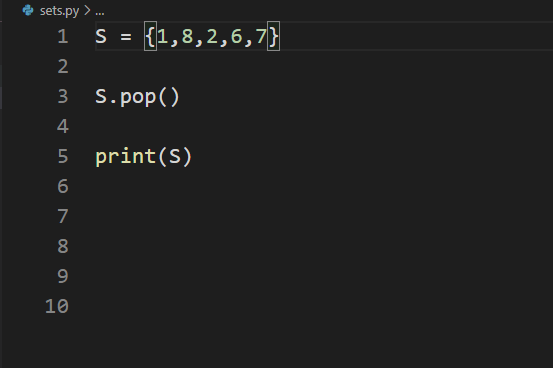
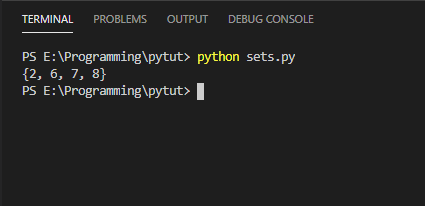
- Set.clear() – It empties the set.
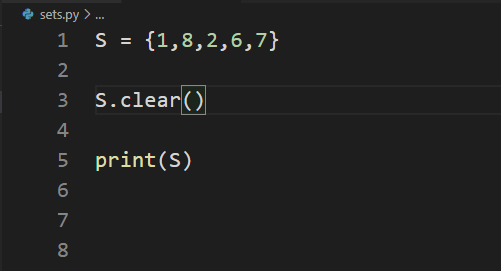
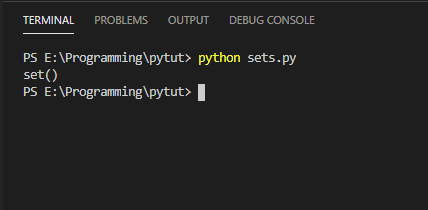
- S.union() – It returns a new set with all items from both sets.
- S.intersection – Returns a set that contains only items in both sets.
Dictionary in Python
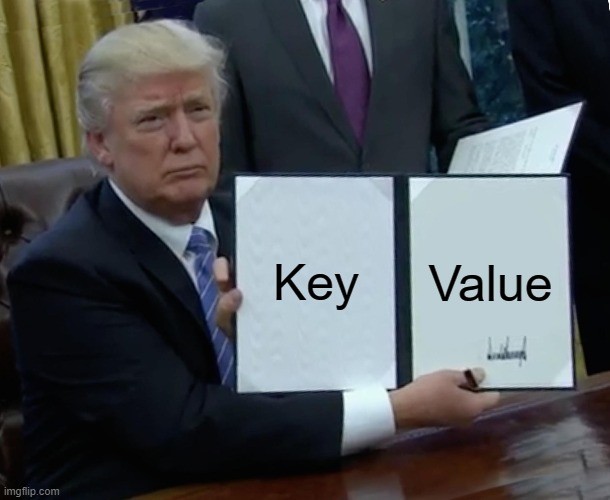
Dictionary is a collection of Key-value pairs in python.
It is like a Dictionary with a word and its specific meaning. Every word has a specific meaning. Every key holds a value. The syntax of Dictionary is like:
MyDictionary = { “Key” : “Value”,
“Kevin”: “Code”,
“Score”: “100”
“list” : [ 1, 2, 9 ] }
Here we made a Dictionary named “MyDictionary” and added items to it. when we print it we can see all the items on the list.

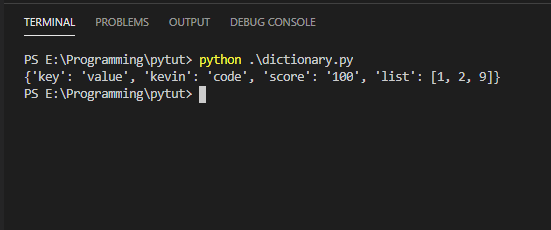
Now we try to access the value of an item using its key and we were able to it. when we use the key “Kevin”, we got the specified value which was “code”. We can do it for other items also.
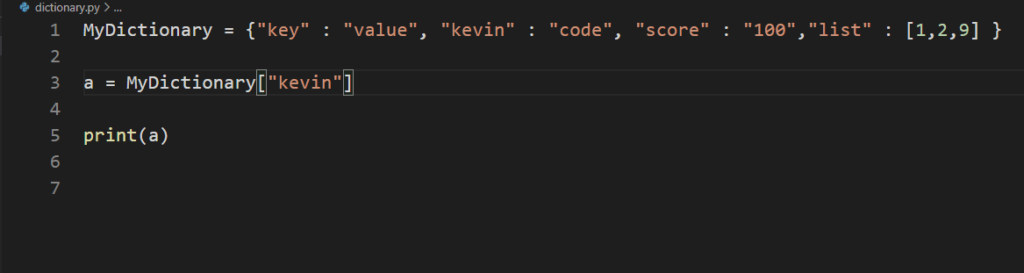
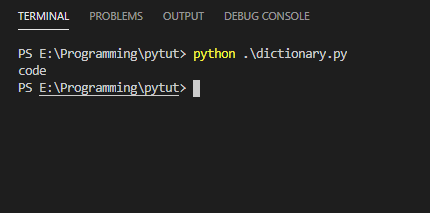
Properties of Python Dictionary
- It is unordered.
- It is Mutable. That means it can be changed.
- It is indexed. That means we can access it via indices.
- It cannot contain duplicate keys.
Dictionary Methods
- Dict.items() – It returns a list of Key-value Tuples.

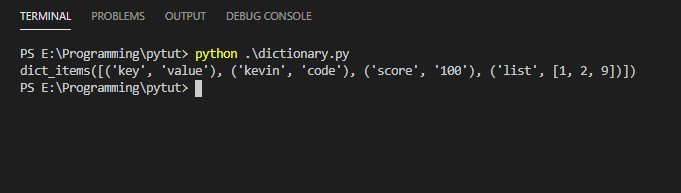
- Dict.keys() – It returns a list containing Dictionary keys.
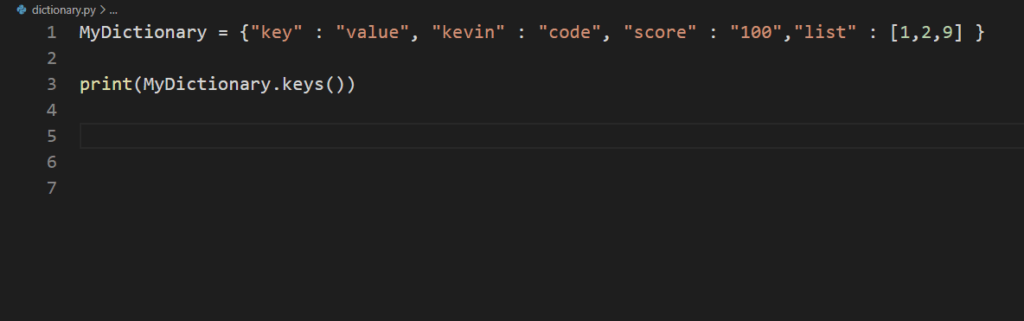
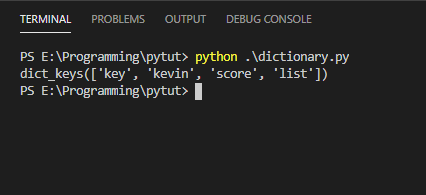
- Dict.update({“friend”: “Sam”}) – It updates the dictionary with the supplied key-value pair.

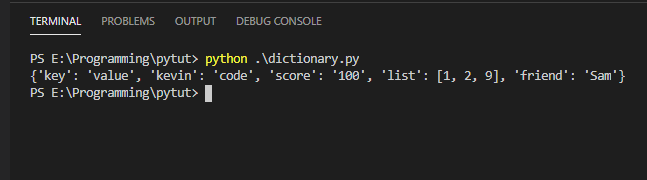
- Dict.get(“kevin”) – It returns the value of the specified keys and the value is returned like “code” is returned here.
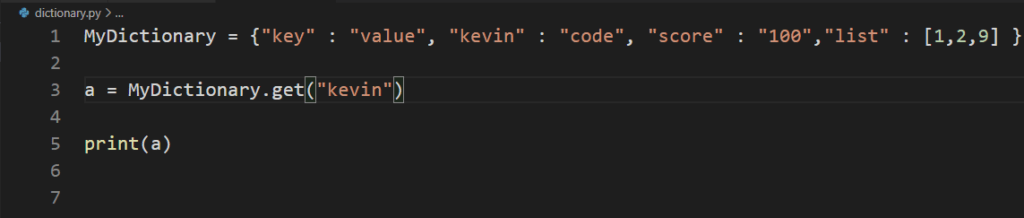
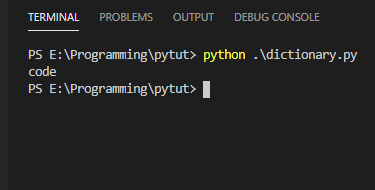
So that’s it for the Dictionary in Python. If you want to check out more of these methods. CLICK HERE. And on that note, this is the end of today’s class. For a revision let’s see what we learned today, we learn what are Sets in python and their properties and methods. Then, we learned about the Dictionary and their functions and how to implement them. So, that’s all for today, this is Dolores Haze, signing off, I will meet you in next one, till then “Happy Coding” and Bye.