What up? My Programming Geeks. So, we have recently completed our Python Course for beginners, in which we have taught you python from scratch to a level to call yourself a python programmer and today’s I thought, why not implement our newly developed skill and make a cool game, and the name of the game is the “The Guess Game”.
So, Guess game huh? Yeah, let me tell you what the game is about.
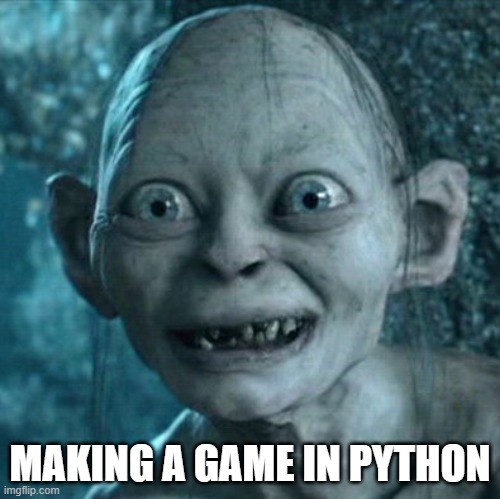
In The Guess Game, the player has to guess a random number between 1 to 100. If he does, he wins otherwise the game continues. We will be developing this game using python. So, let’s get started.
Ingredients for making the game
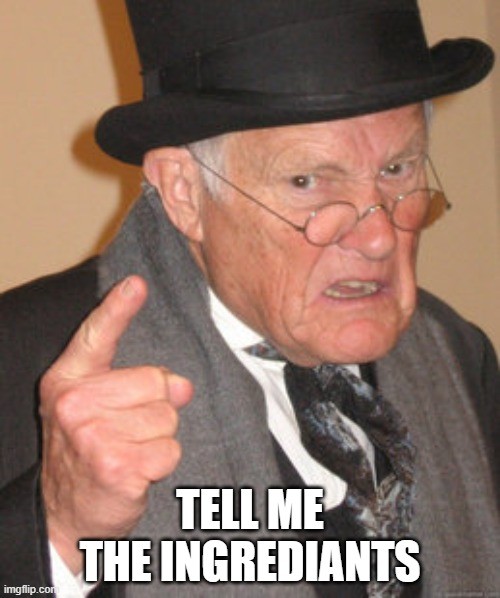
In order to start making a delicious meal, we should know the recipe and before the recipe. We must know, the ingredients used. With that say, we have to do these things using python:
- Generate a Random number.
- Let the user enter a number.
- Play the game until the user wins.
- Once the user wins, tell him his score in the form of guesses.
- Develop a Hint.
- Create a Highscore system in order to record the maximum score.
So, that’s what we need to do in our program. Each point is used in code and will describe in there. So, let’s jump right into the making.
The making of The Guess Game
import random #importing random module import pyfiglet # importing Pyfiglet module ascii_banner = pyfiglet.figlet_format("THE GUESS GAME") # ascii banner using Pyfiglet print(ascii_banner) guess = 0 #initialize guess to 0 while True: try: number = int(input("Guess the number: ")) num = random.randint(1, 5) #using randint function from random guess = guess + 1 if number == num: print( f"You have guessed the number in {guess} guesses. Congrats !!!") break else: print("Try again !!!") if number > num: print(f"Hint: Enter a number smaller than {number}!!!") else: print(f"Hint: Enter a number greater than {number}!!!") except Exception as e: print(f"Error: {e}: Please Enter a number") with open("hiscore.txt", "r") as f: #creating hiscore part score = f.read() score = int(score) if guess < score: with open("hiscore.txt", "w") as f: f.write(str(guess)) print("You made a HIGHSCORE !!!") input() #extra input to hold program after exit
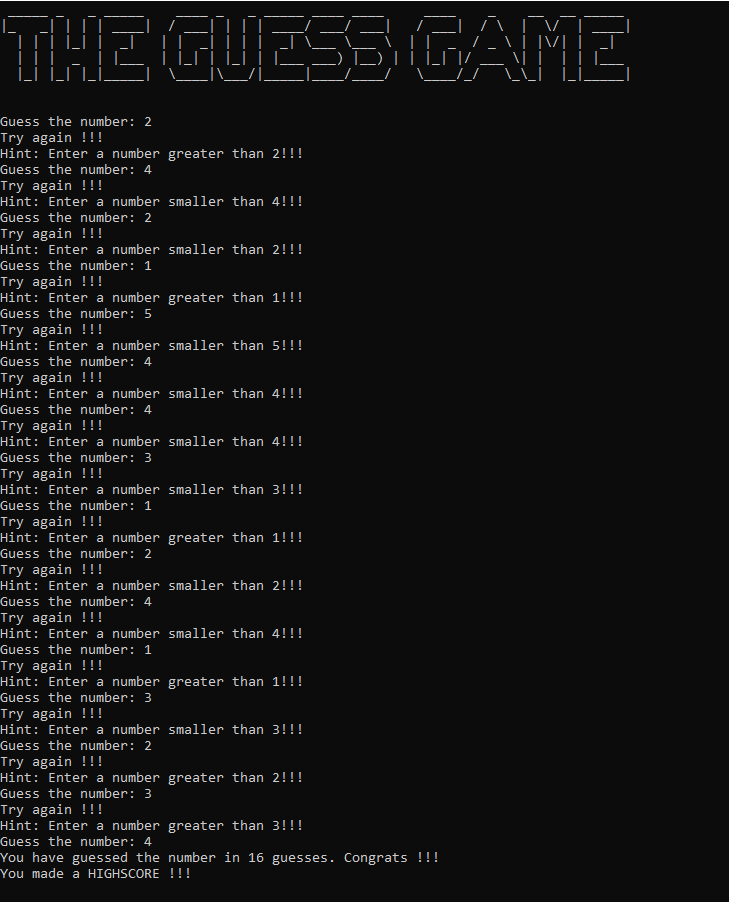
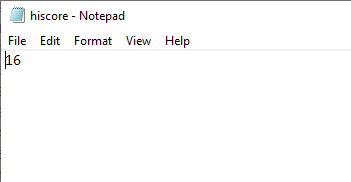
Let’s start from the first ingredient, We generate a Random number using the Random module. Then we use it using Random.Randint(1,100), that will generate a random number between 1 to 100.
Then, moving to the second point, we use the input function to let the user enter a number of his choice, which is apparently his guess.
The third point says that play the game until the user wins. We know about loops right, so we use While loop here and set the While loop to True, which means it will run forever. The condition here to win is when the user guess is equal to the random number, the user wins. The loop will break once the user wins, this happens due to the use of the break statement.
For score, we first initialize guess = 0, then we increment it after every input. Plus, when we win, we display it using f strings, Eazy-Peazy.
Next, we have to develop the hint, we do it using a condition to check if the number entered is greater than the random number, tell the user to enter a smaller number otherwise enter a greater number.
Next and most important, create a Highscore table. We can do that by making a text file named “hiscore.txt” and maintaining and changing the hiscore in it if the condition satisfies. After every win, the guess is checked with the score in the text file. If it is greater than okay, if it is lesser, it’s the new high score and it will be updated. This was possible due to file handling in python, in which first we open the file in read mode and if condition is true then in write mode.
One more thing here is the try and except clause. This is used to show an error and avoid the program to crash due to an illegal input or something like that. You have studied these concepts in the course, if you haven’t checked the course, do it now, CLICK HERE. For Downloading Source code and program, Download it from here:
So, that was it. We develop a really cool game using python and the best thing is that it involves many concepts of Python programming. it is a very good project to get your hands dirty and have a hands-on experience. Plus, It’s easy to make if your concepts are clear. So, on that note, that’s all for today, this is Dolores Haze, signing off, I will be back with other interesting projects, till then, “Happy Coding” and Bye.