Hello and Welcome to Python Programming for beginners, where if teach you Python from Scratch. It’s been a long journey and we have come far ahead with our python Programming class. In the last class, we see Loops and Jump Statements. If you haven’t checked them out, CLICK HERE and if you had, let’s continue with today’s class.
So, the sole agenda of today’s class is “Strings in Python”. So, let’s jump into it and see what it holds.
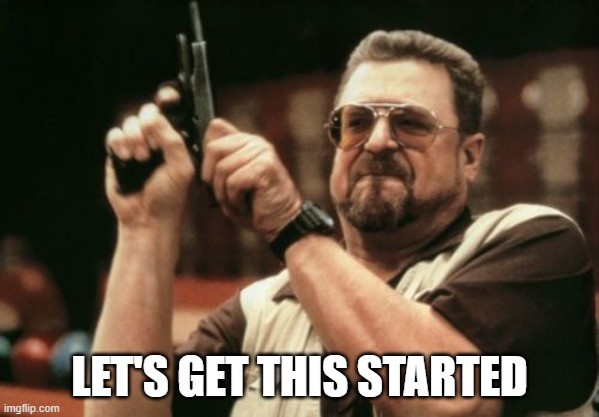
Strings in Python
String is a data type in Python. String is a sequence of characters enclosed in quotes.
We have already seen, String data type in Python in our “Variables and data types” class. Now, we will see how we can do string manipulation.
We can primarily write a string in three ways.
- Single quoted string – name = ‘Peter’
- Double quoted string – name = “Peter”
- Triple quoted-string – name = ‘’’Peter’’’
On that note, Let’s write a program that will take a name as input by the user and display a greeting message like “Good Morning”.
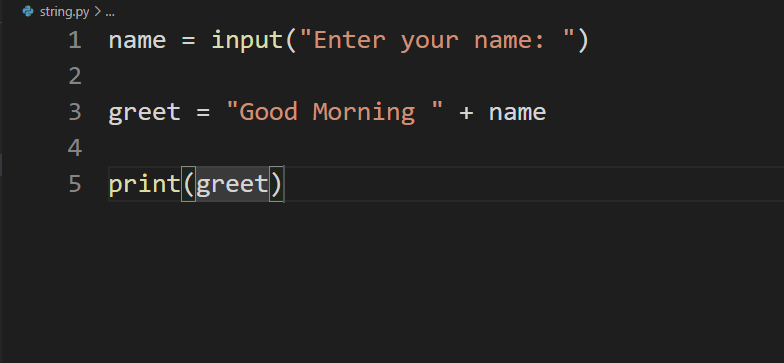
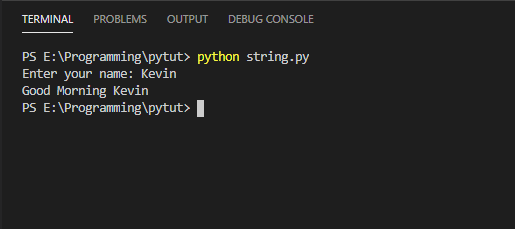
Here we take a name as input by the user using the input function, then we write a string greet and concatenate the name with it, simply by doing “Good Morning” + name. that’s it and we print it, easily.
We can also use f string to do this, f string is a great way to print something, here let me show you how.
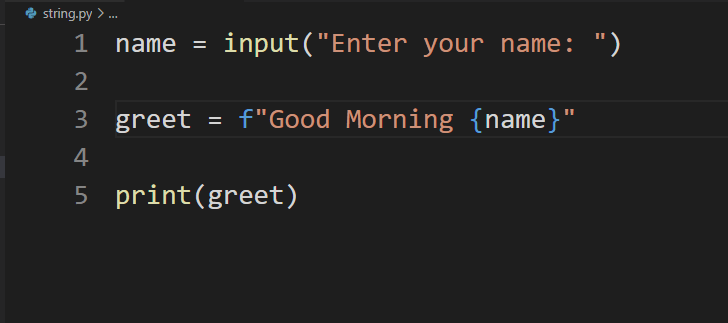
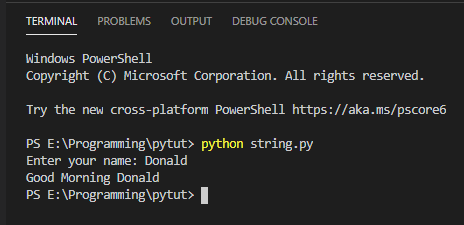
Here we write f”string text {variable_name}”. This is a great way to work with string as it takes the variable name and makes your life easier.
Strings Slicing
A string in python can be sliced for getting a part of the string. For example, there is a string name that has the value “Kevin”.
Name = “Kevin”
The length of the string is 5. We have seen loops and in python programming, we know the index starts with 0.
In the same way, the index in string starts from 0 to (length – 1) in python. That means here it will go from 0 to 4, where K is at 0 and n is at 4.
To slice a string, we use the following syntax.
SL = name [index_start : index_end]
Here, Index_start is included whereas index_end is not included.
Here index_start is 0 and index_end is 3. So with that, we can write the above string like this.
Here you can see that it goes from 0 to (3-1). And we got the answer “Kev”. So, that’s how slicing works.
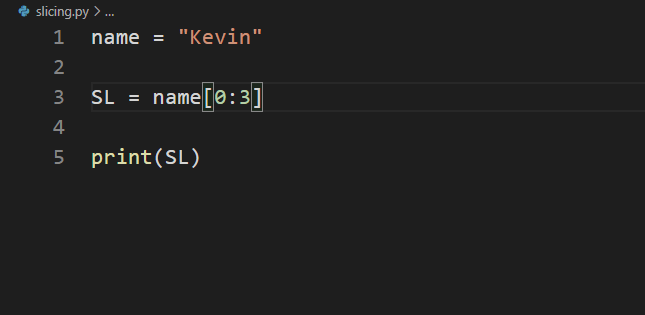
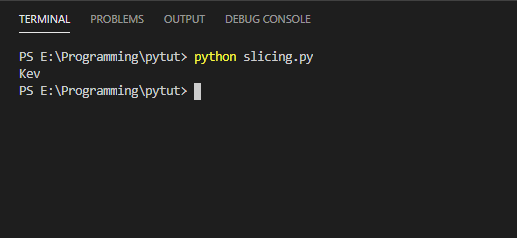
Negative Indices – In slicing, we can also use negative indices, though it is not preferable by most of the people but you can do it still. Here -1 is length-1 and so on till -5. As there are 5 indices in Kevin. So, n is -1 and K is -5. Try it out yourself.
Slicing with skip value – We can provide a skip value as a part of our slice. For example, if have a string called word which holds a word called amazing like this.
Word = “amazing”
Here, it slices the word from 1 to 8 with a skip value of 2. So we got “mzn” as an answer. You can use it in various places with strings.
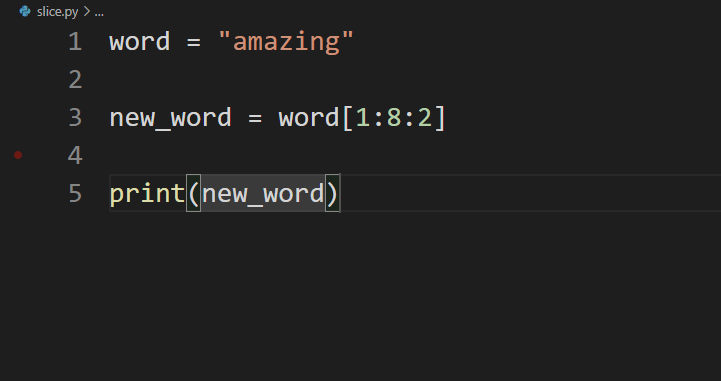
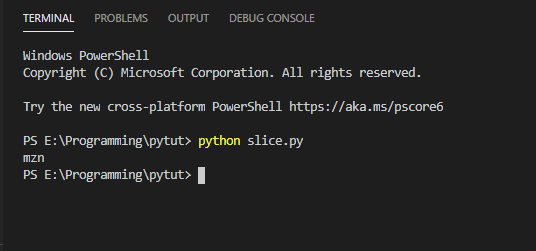
Some other advanced slicing techniques are:
Word = “amazing”
- Word[ : 7] à It means same as Word[0:7]. It prints “amazing”.
- Word[0: ] à It means same as Word[0:7]. It also prints “amazing”.
String Functions in Python
There are some in-built string functions in python, which we can use. Let’s see some of them.
- Len() function – The len() function in python, gives us the the length of the string. Ex:
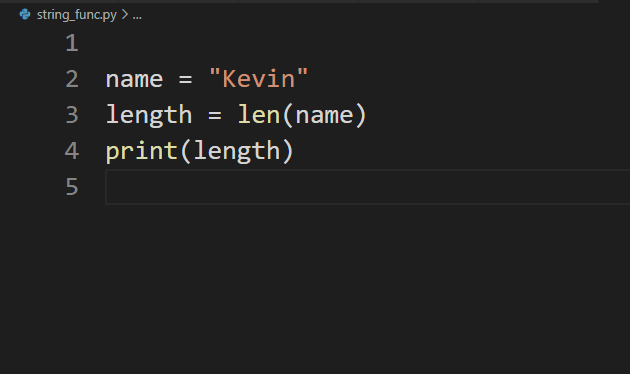
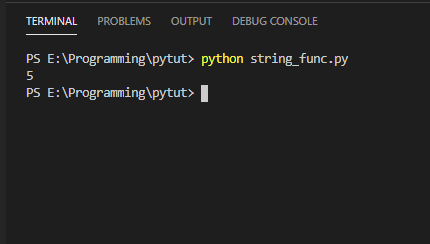
- String.endswith(“vin”) – This function tells whether the variable string ends with the string “vin” or not. If string is “Kevin”, it returns True for “vin”, since Kevin ends with “vin”.
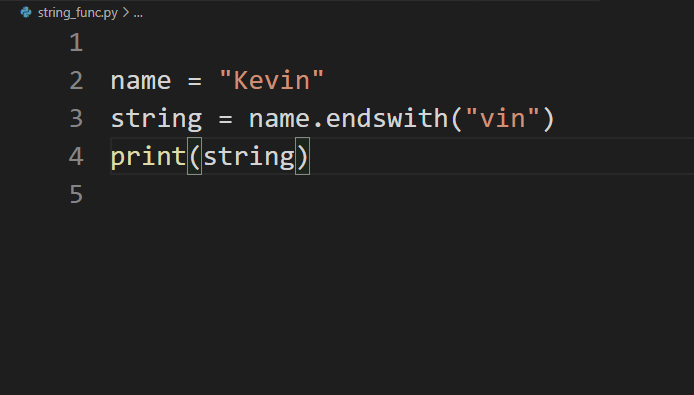
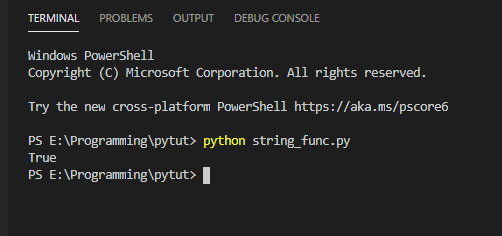
- String.count(“c”) – Counts the total number of occurrences of any character.
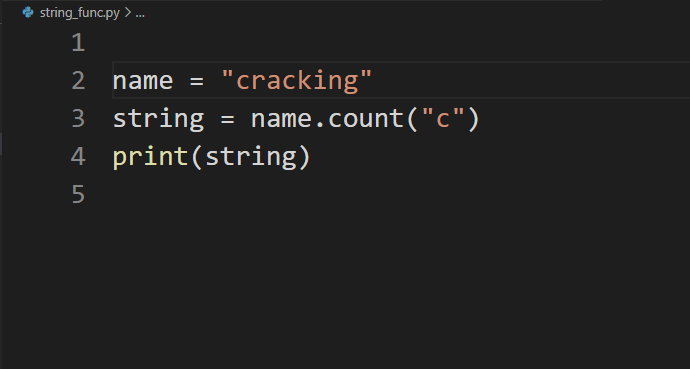
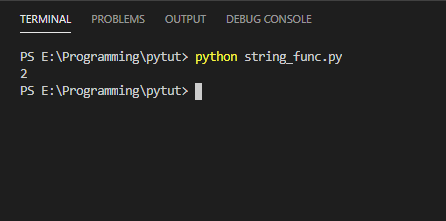
- String.capitalize() – This function capitalizes the first character of a given string.
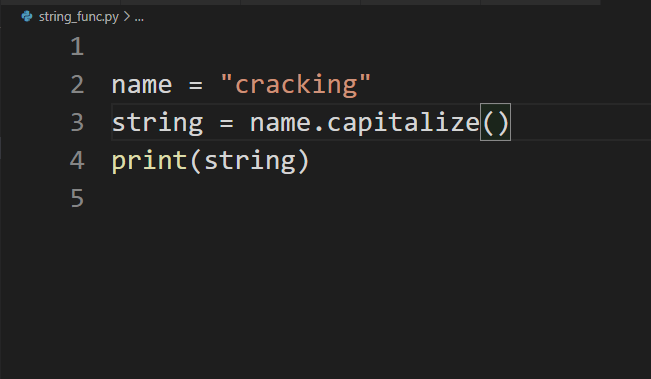
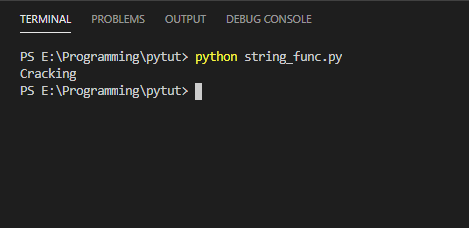
- String.find(word) – This function finds for the given word and returns the index of first occurrence of that word in the string.
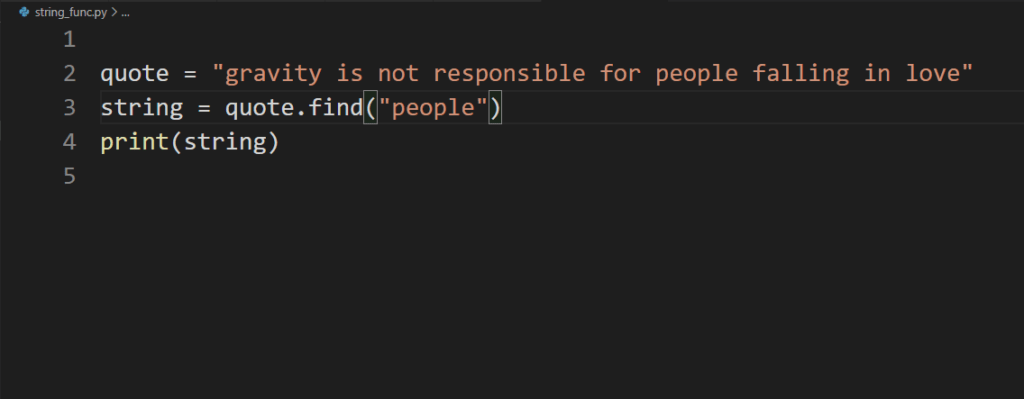
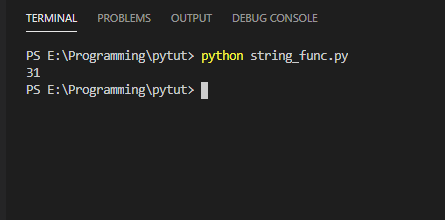
- String.replace(oldword, newword) – This function replaces the oldword with newword in the entire string.
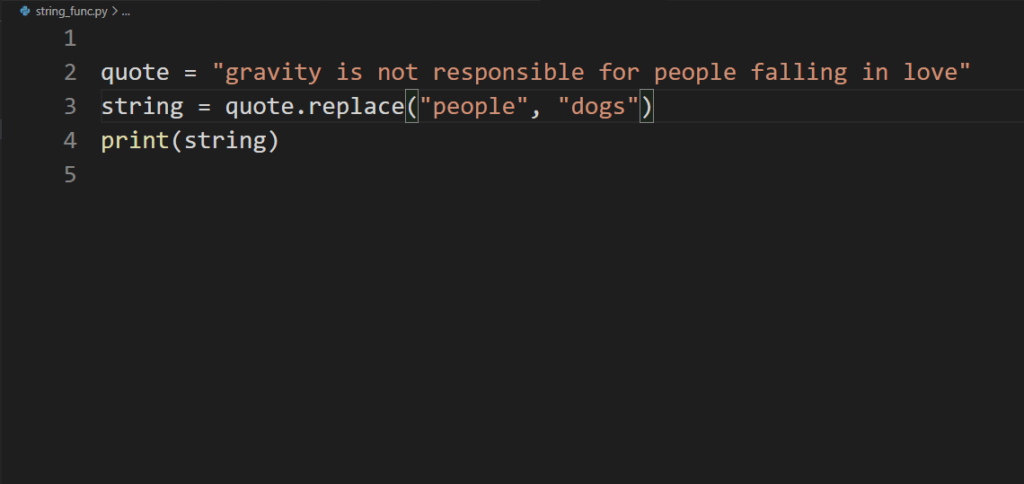
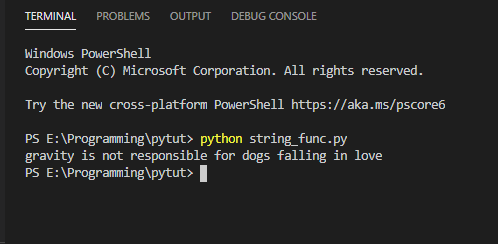
Escape sequences in Python
Sequence of characters after backslash “ \ ”.
Escape sequence character comprises of more than one character but represents one character when used within string. Some examples of escape sequences characters are: “\n” used for a new line, “\t” used for a tab, “ \’ “ for single quote, “ \\” for backslash, and there are more.
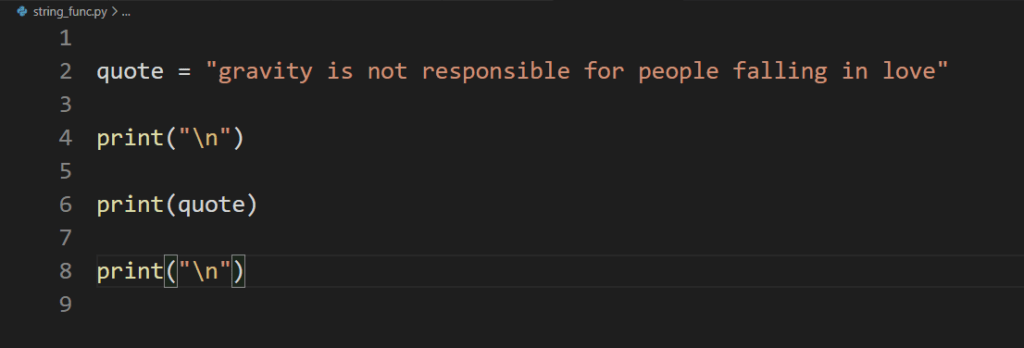
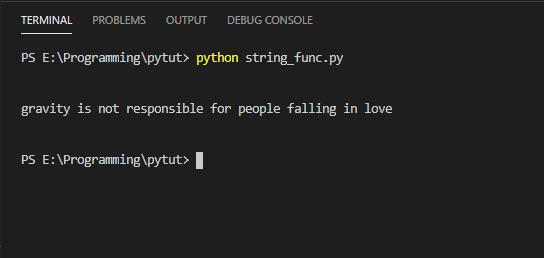
So, that all for today’s class. For a revision, what we have learned today, we have seen what are strings in Python, what is slicing and how to perform slicing on strings, what are string functions, and escape sequences in the end. This is one of the most important classes as you will be using strings everywhere. So, on that note, that’s all for today, this is Dolores Haze, signing off, I will meet you in next one, till then “Happy Coding” and Bye.